Assignment 4
Data Modeling
Note: some changes have been made since Assignment 3 to accomodate implementation.
Concept 1 - User
Purpose: Save user information over various sessions on the application
Principle: Whenever they use the app, user signs in with a username and password
State:
- registered: set User
- username, password: User -> one String
Actions:
- register ()
- authenticate (username: one String, password: one String, out b: one Boolean)
- login (username: one String, password: one String, out u: User)
- delete ()
Concept 2 - Post
Purpose: Upload content to the platform
Principle: Users can create and publish posts containing media such as text, image, video, etc.
State:
- content: set String, set Media (Media is a concept that denotes simple photos or video that may be uploaded as part of a post)
Actions:
- publish (title: one String, out p: Post)
- edit (s: set String)
- upload (v: set Media)
- remove (p: one Media)
- delete ()
Concept 3 - Savour
Purpose: Keep and organize (recipe) posts for future reference
Principle: When a user saves a post, a link to the post is added to some easily accessible page that the user can refer back to later
State:
- saved: set Post
Actions:
- save (p: Post)
- unsave (p: Post)
Concept 4 - Tag and Filter
Purpose: Organize posts with overlapping
Principle: Users can assign tags to their own posts and to posts that they save; users can only assign public tags to their own posts, but they can assign private tags to any post
State:
- tags: set String
- tagged: Post -> one Tag,
Actions:
- create (tag_name: one String, private: one Boolean)
- add (p: Post, t: Tag)
- remove (p: Post, t: Tag)
- find (ts: set Tag, out ps: set Post)
Concept 5 - Post Notes
Purpose: Duplicate posts (recipes) and customize them in your own save folders
Principle: Save only the desired content of a post and add personal comments/notes on that post in your records
State:
- notes: Post -> one String
Actions:
- create (s: one String, out p: Post)
- edit (s: one String)
Concept 6 - Ingredient Match (specialized search)
Purpose: Given a set of keywords (i.e. ingredients), find posts that contain those keywords
Principle: User enters a list of ingredients, and a list of posts including all of those ingredients is generated
State:
- query (one String -> set Post)
Actions:
- find (ss: one String, out ps: set Post)
Concept 7 - Featured Posts
Purpose: Highlight a subset of posts based on some criteria (tags, popularity, etc.)
Principle: For a given time cadence (i.e. daily, weekly), a new set of posts is chosen by some algorithm and is featured in the application via display or promotion
State:
- featured: set Post
Actions:
- set_theme (s: String)
- feature (p: Post)
- unfeature (p: Post)
Diagrams
Dependency Diagram
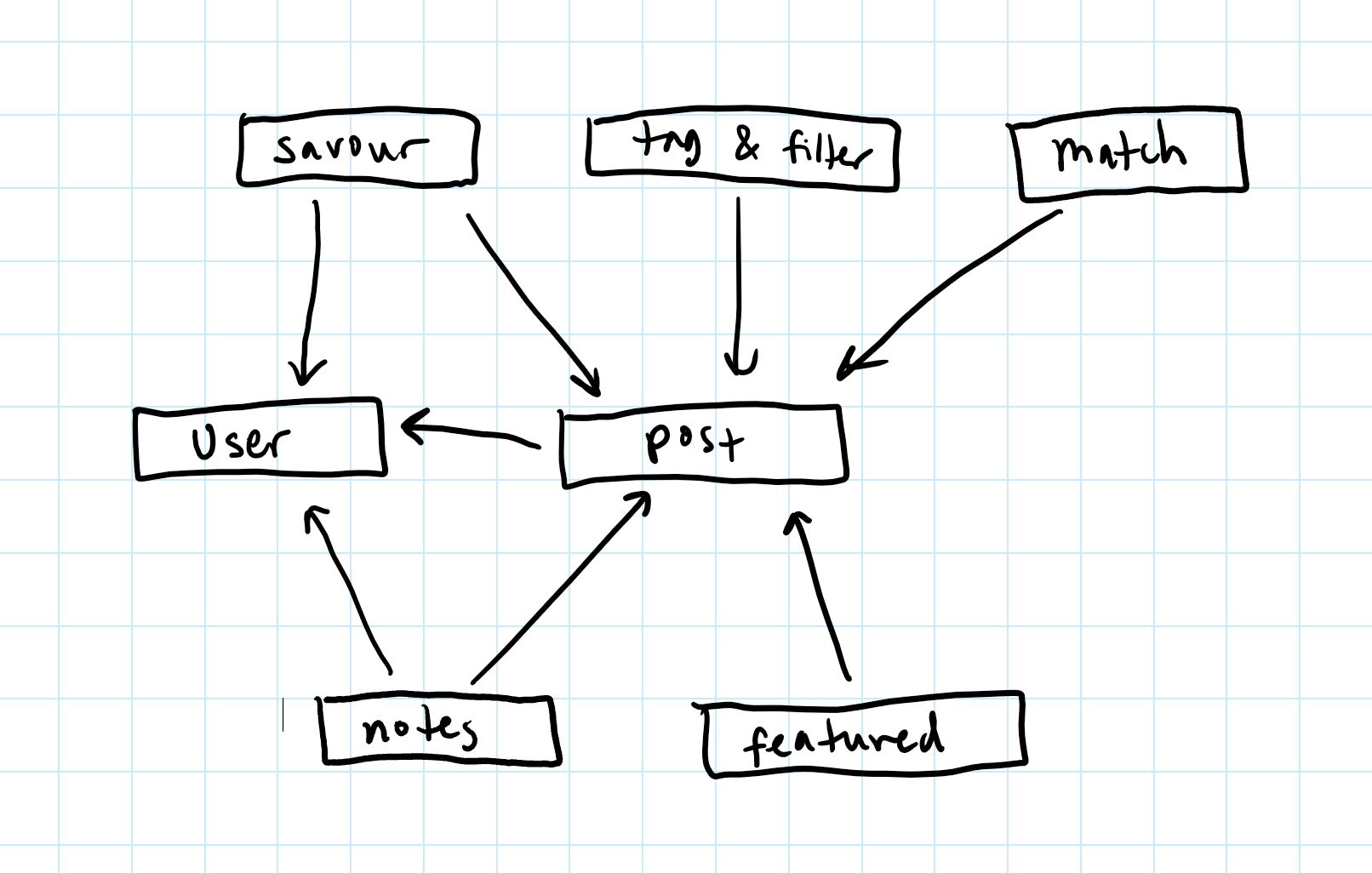
Data Model Diagram
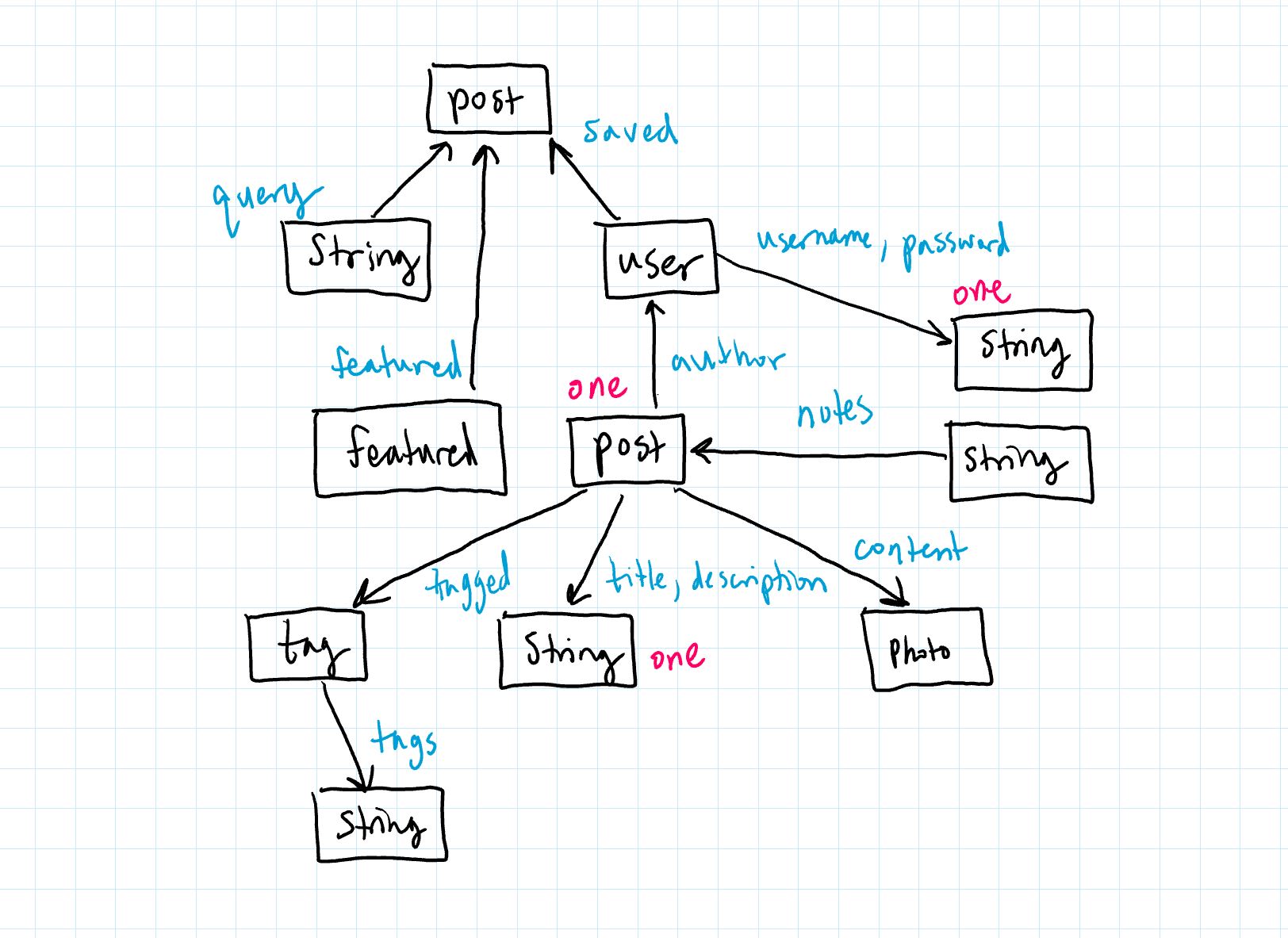
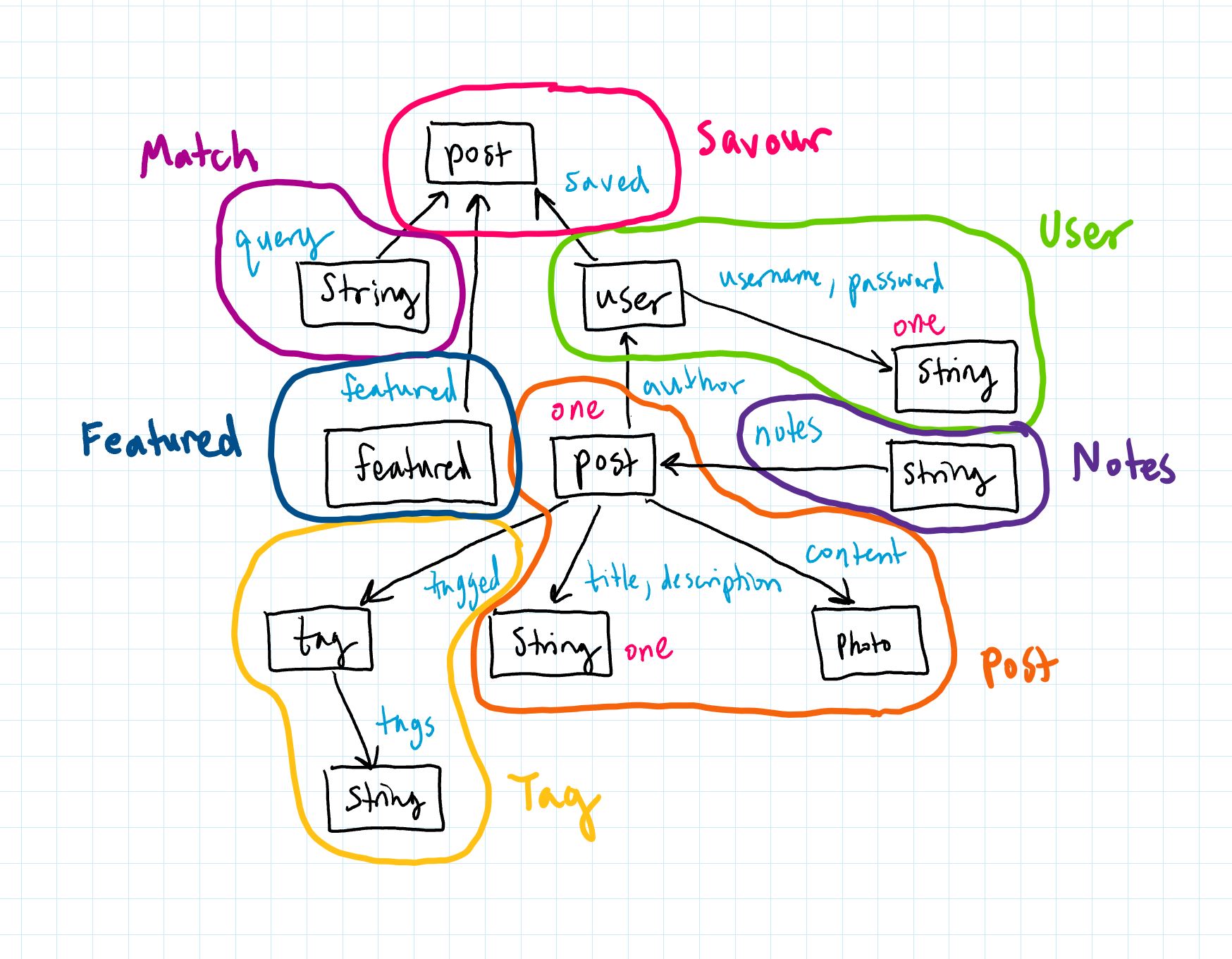
Demo Links
Backend Repository
https://github.com/grace-j784/backend-starter
Vercel Deployment
https://backend-starter-cyan.vercel.app/
Design Reflection
During implementation, some details in the concept actions were updated to make structuring the backend easier. In addition, some concepts were merged into one module to reflect synchronizations and partition concepts into groups where concepts interact more with each other and are more integrated. From a UI perspective, one question I considered when implementing my concepts was how to design the routes and entries in the utils file. For example, with tag, I wasn't sure how having separate interfaces for adding public vs. private tags would compare to combining both into one interface with an input for public vs. private. The latter seemed more likely to be error-prone or confusing, but I ended up choosing this method in favor of less repetitive code and more compact design. In terms of design choices around functionality, I considered a couple of different options for implementing tag, and I ultimately decided to keep a database that stores each assignment of a tag to post. While this could lead to repetitive tag-post entries, I decided that this would be okay for users, since for every assignment they add, they can lookup and delete it. The benefit of this is that the tag code can be cleanly modularized, and there is no need for nested databases. Finally, I made the choice to turn post notes into an attribute of saved. Since any post with notes is automatically saved per my previous design, it made sense to integrate the two concepts, and it keeps the related functionalities in one place without bloating the backend with too many files. Overall, my choices were made to simplify the backend code. While this loses some elegance in terms of UI/UX, it makes the backend easy to parse and actions relatively straightforward to understand.